Collection module
đ Descriptionâ
The Collection
module contains classes and controls to define item collections in the builder. Item collections are used to enable the user to create lists with items of a certain type. For example, options in a dropdown list.
See the Collections guide for more information.
đ Classesâ
đē Previewâ
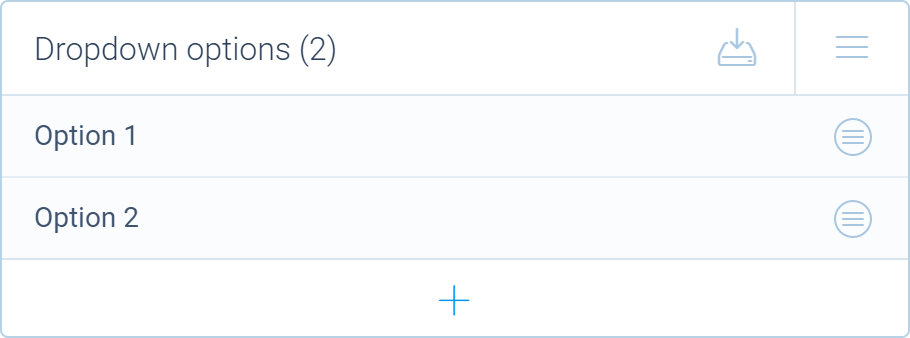
âļī¸ Functionsâ
đ§ find
â
Finds the collection that serves the list of possible values for a certain slot. For example, let's say you have a dropdown block with a collection that contains all the possible dropdown options and a single slot that holds the selected option for the dropdown. This function can retrieve a reference to the collection Provider
for that slot.
Signatureâ
find(slot: ISlotIdentifier): ICollectionSupply | undefined
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
slot | ISlotIdentifier | No | Specifies the slot to find the collection for. |
Return valueâ
Returns a ICollectionSupply
object or undefined
if the collection was not found.
đ§ get
â
Retrieves the collection with the specified property name from a NodeBlock
.
Signatureâ
get(block: NodeBlock, name: string): Provider | undefined
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
block | NodeBlock | No | Reference to the node block to search in. |
name | string | No | The name of the property that holds the collection. |
Return valueâ
Returns a reference to the Provider
instance or undefined
if the collection was not found.
đ§ of
â
Creates a new collection of the supplied item type class. The type class needs to be derived from the Item
class. This function returns a reference to the Provider
that provides access to the collection in the builder.
Collections are automatically saved to and retrieved from the form definition (as if the collection field was decorated with the @definition
decorator).
Signatureâ
of(constructor: typeof Item, reference: NodeBlock | ConditionBlock | Item): Provider
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
constructor | typeof Item | No | Specifies the type of items for the collection. |
reference | NodeBlock | ConditionBlock | Item | No | A reference to the parent instance that holds the collection. This reference is exposed to the ref property of the Item . |
Return valueâ
Returns a reference to the Provider
instance.
Exampleâ
import { _, tripetto, definition, name, Collection, NodeBlock } from "@tripetto/builder";
// Let's define an item type that holds an option for a dropdown list
class DropdownOption extends Collection.Item<Dropdown> {
@definition
@name
name = "";
}
@tripetto({
type: "node",
identifier: "dropdown",
label: "Dropdown",
icon: "data:image/svg+xml;base64,PHN2ZyAvPg=="
})
class Dropdown extends NodeBlock {
// Create a collection of dropdown options
options = Collection.of<DropdownOption, Dropdown>(DropdownOption, this);
}
âī¸ Interfacesâ
đ IProperties
â
Describes the interface for declaring a collection card using the collection
method of the EditorOrchestrator
class for defining editor panels.
Type declarationâ
interface IProperties { collection: Provider; title: string; banner?: string;Optional sorting?: "ascending" | "descending" | "manual";Optional placeholder?: string;Optional emptyMessage?: string | { message: string; height: number; };Optional icon?: boolean | string;Optional editable?: boolean;Optional autoOpen?: boolean;Optional allowAutoSorting?: boolean;Optional allowCleanup?: boolean | string;Optional allowVariables?: boolean;Optional allowFormatting?: boolean;Optional allowImport?: boolean;Optional allowExport?: boolean;Optional allowDedupe?: boolean;Optional showAliases?: boolean | ((item: Item) => string | undefined);Optional showScores?: boolean | ((item: Item) => number | string | undefined);Optional markdown?: MarkdownFeatures;Optional menu?: MenuOption[] | (() => MenuOption[]);Optional width?: number;Optional indicator?: (item: Item) => string | undefined;FunctionOptional onAdd?: (item: Item) => void;EventOptional onRename?: (item: Item) => void;EventOptional onReposition?: (item: Item) => void;EventOptional onResize?: (collection: Provider) => void;EventOptional onDelete?: (item: Item) => void;EventOptional onOpen?: (item: Item) => void;EventOptional onClose?: (item: Item) => void;EventOptional }
đˇī¸ allowAutoSorting
â
Specifies if the option to automatically sort the list is shown (enabled by default).
This only applies to collection cards with sorting
set to manual
.
Typeâ
boolean
đˇī¸ allowCleanup
â
Specifies if the option to delete all unnamed items is shown (enabled by default). When a string is supplied the option is enabled and that string is shown as menu option to execute the cleanup.
Typeâ
boolean | string
đˇī¸ allowDedupe
â
Specifies if the deduplication function is allowed. Deduplication deletes items with the same name.
Typeâ
boolean
đˇī¸ allowExport
â
Specifies if the bulk item exporter is allowed.
Typeâ
boolean
đˇī¸ allowFormatting
â
Specifies if markdown formatting is rendered in the list.
Typeâ
boolean
đˇī¸ allowImport
â
Specifies if the bulk item importer is allowed.
Typeâ
boolean
đˇī¸ allowVariables
â
Specifies if the use of variables (recalling values) is supported.
Typeâ
boolean
đˇī¸ autoOpen
â
Specifies whether to open the editor panel of new items when they are added.
Typeâ
boolean
đˇī¸ banner
â
Specifies a banner for collection card. The banner is shown above the card.
Typeâ
string
đˇī¸ collection
â
Reference to the collection Provider
instance.
Typeâ
đˇī¸ editable
â
Specifies if the name of the items can be edited in the list by double tapping on the name.
Typeâ
boolean
đˇī¸ emptyMessage
â
Specifies the message that is shown when the collection is empty.
Typeâ
string | {
/* Specifies the message to show. */
message: string;
/* Specifies the height of the collection card in pixels when the message is shown. */
height: number;
}
đˇī¸ icon
â
Specifies if an icon is shown for each item (a default icon can be supplied). The Item
property that is decorated with the @icon
decorator is used as icon for the item.
Typeâ
boolean | string | SVGImage
đˇī¸ indicator
â
Specifies an indicator for each Item
that is shown in the collection card.
Typeâ
(item: Item
) => string | undefined
đˇī¸ markdown
â
Specifies the markdown features that are enabled when allowFormatting
is set to true
.
Typeâ
đˇī¸ menu
â
Specifies a menu that is shown when the user wants to add something to the collection.
Typeâ
MenuOption[]
| (() => MenuOption[]
)
đˇī¸ placeholder
â
Specifies the placeholder for unnamed items.
Typeâ
string
đˇī¸ showAliases
â
Specifies to show aliases. If this property is set to true
it will use the Item
property that is decorated with the @alias
decorator. A function can be supplied to return an alternative alias for an Item
.
Typeâ
boolean | ((item: Item
) => string | undefined)
đˇī¸ showScores
â
Specifies to show the score of an item. If this property is set to true
it will use the Item
property that is decorated with the @score
decorator. A function can be supplied to return an alternative score for an Item
.
Typeâ
boolean | ((item: Item
) => number | string | undefined)
đˇī¸ sorting
â
Specifies the collection sorting type (defaults to manual
). In manual mode, the user can rearrange the list using drag and drop.
Typeâ
"ascending" | "descending" | "manual"
đˇī¸ title
â
Title for the collection card. The title is shown inside the collection card.
If you want to have a title above the card, use the banner
property.
Typeâ
string
đˇī¸ width
â
Specifies the width if the item editor panel. Can be overruled per item with the @width
decorator.
Typeâ
number
đ onAdd
â
Invoked when an item is added to the collection.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the new item. |
đ onClose
â
Invoked when the editor panel of an item is closed.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the item. |
đ onDelete
â
Invoked when an item is removed from the collection.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the deleted item. |
đ onOpen
â
Invoked when the editor panel of an item is opened.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the item. |
đ onRename
â
Invoked when the name of the item is changed.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the changed item. |
đ onReposition
â
Invoked when the position of the item is changed.
Signatureâ
(item: Item) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
item | Item | No | Reference to the changed item. |
đ onResize
â
Invoked when the number of items in the collection has changed.
Signatureâ
(collection: Provider) => void
Parametersâ
Name | Type | Optional | Description |
---|---|---|---|
collection | Provider | No | Reference to the collection provider. |
đ ICollectionSupply
â
Describes the interface for a collection supply returned by the find
function.
Type declarationâ
interface ICollectionSupply { name: string;Readonly collection: Provider;Readonly origin: boolean;Readonly sole: boolean;Readonly }
đˇī¸ collection
â
Contains a reference to the collection Provider
instance.
Typeâ
đˇī¸ name
â
Contains the name of the collection property in the NodeBlock
derived class.
Typeâ
string
đˇī¸ origin
â
Specifies if the collection is the origin for the slot. This means the slot is created/maintained by the collection. For example, you have a multiple choice block where the choices are maintained using a collection. For each choice, a
Typeâ
boolean
đˇī¸ sole
â
Specifies if the collection is the sole supplier of values for the slot. For example, if your slot is used for a fixed dropdown list and the dropdown options are supplied by the collection, that collection is the sole supplier of possible values for the slot. If your slot is used for a text field with autocomplete suggestions and the collection is the supplier for the suggestions, the collection is not the sole supplier, because the respondent can also specify another value that is not in the collection list.
Typeâ
boolean