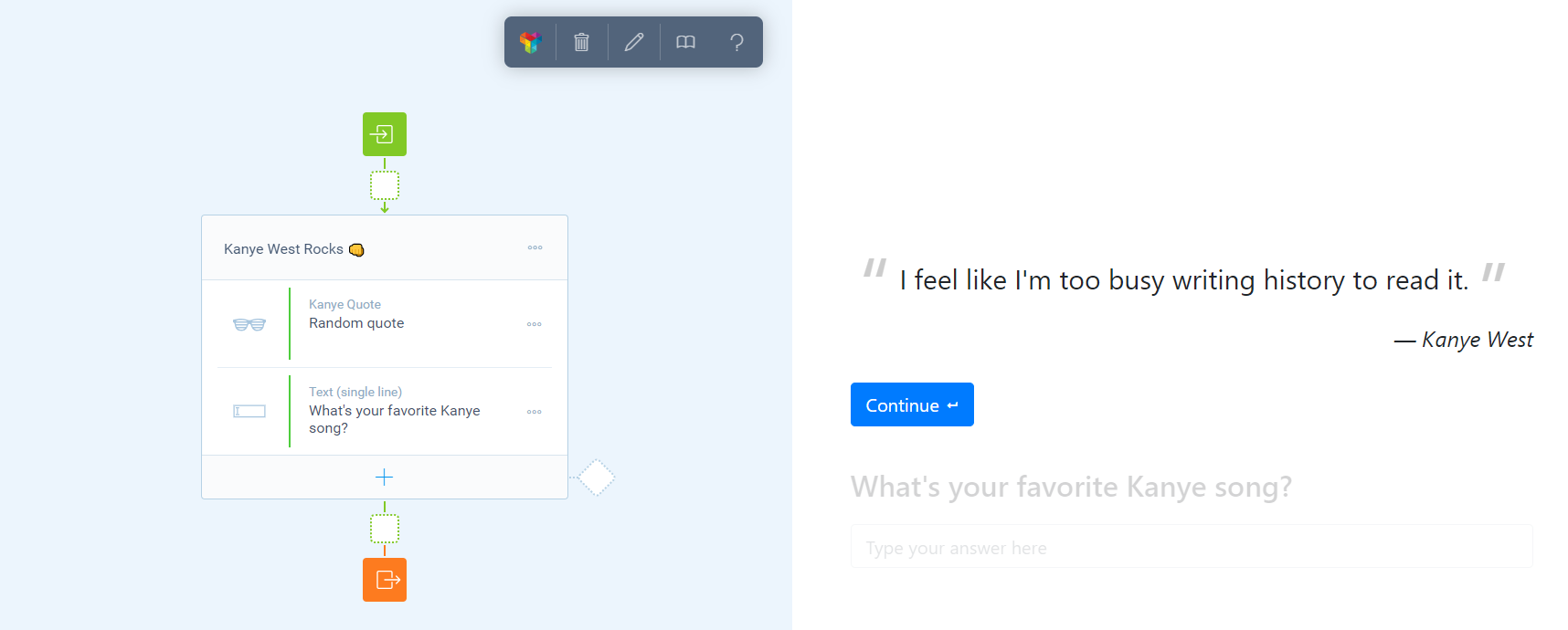
Add some Kanye genius to your survey (tutorial on integrating an API)
How to integrate an API in your survey with Tripetto.
❌ This is a deprecated SDK blog article
Please note that the SDK concepts and code snippets in this article are about a deprecated version of the Tripetto SDK. In the meantime the SDK has evolved heavingly. For up-to-date SDK documentation, please have a look at the following links:
Extend your survey with custom question types
When designing a survey you usually have a number of basic question types to choose from like Number, Dropdown or Star Rating. Some vendors make more complex question types available like Matrix or Payment.
With Tripetto you can build your own question types. This brings endless opportunities to enrich your survey and collect more user data. In this article I’ll demonstrate how easy it is to create a question type that connects to an external API. This example isn’t a question actually, but it shows a random quote from Kanye West any where you need his genius.

Concept
Tripetto distinguishes itself with the way it visually presents the flow of a survey in the Editor. When building a custom block (Tripetto uses blocks as a synonym for question types) you have to define how your custom block exposes itself in the editor and which properties you can edit.
Another part of the custom block is how it represents itself in the Collector. The collector is where your respondents file your survey. Tripetto gives you absolute freedom how to display your survey, but there are some examples to give you a head start.
This example is based on the Conversational UX demo with React.
Editor
To create a custom block in the editor you have to implement the NodeBlock
abstract class, prefixed with the @tripetto
decorator to supply (meta) information about the block.
🚧 Warning: Deprecated SDK code snippets and packages
Please note that the below code snippets and used packages are about a deprecated version of the Tripetto SDK. In the meantime the SDK has evolved heavingly. For up-to-date SDK documentation, please have a look at the following links:
import { NodeBlock, Slots, slots, tripetto } from "tripetto";
import * as ICON from "./kanye.svg";
@tripetto({
type: "node",
identifier: "kanye",
label: "Kanye Quote",
icon: ICON
})
export class Kanye extends NodeBlock {
@slots
defineSlot(): void {
this.slots.static({
type: Slots.String,
reference: "kanye-quote",
label: "Kanye quote"
});
}
@editor
defineEditor(): void {
this.editor.name(false, false, "Name");
this.editor.visibility();
}
}
Slots are a fundamental part of a custom block, because they define how response data is stored. In this example I’ve chosen for a single static slot of type Slots.String
where I can store the random quote that was presented to the respondent.
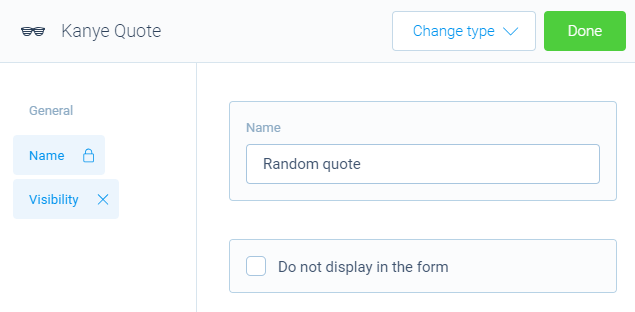
Collector
The collector is where the real API magic happens! In the editor we’ve described the way our custom block can be managed. In the collector we’ll describe how it looks and how it works. Since this example is based on React and Bootstrap, this is where these frameworks come together with Tripetto.
🚧 Warning: Deprecated SDK code snippets and packages
Please note that the below code snippets and used packages are about a deprecated version of the Tripetto SDK. In the meantime the SDK has evolved heavingly. For up-to-date SDK documentation, please have a look at the following links:
import * as React from "react";
import * as Tripetto from "tripetto-collector";
import * as Superagent from "superagent";
import { IBlockRenderer } from "collector/helpers/interfaces/renderer";
import { IBlockHelper } from "collector/helpers/interfaces/helper";
import "./kanye.scss";
const DEFAULT_QUOTE = "I feel like I'm too busy writing history to read it.";
@Tripetto.block({
type: "node",
identifier: "kanye"
})
export class KanyeBlock extends Tripetto.NodeBlock implements IBlockRenderer {
readonly quoteSlot = Tripetto.assert(this.valueOf<string>("kanye-quote"));
constructor(node: Tripetto.Node, context: Tripetto.Context) {
super(node, context);
Superagent
.get("https://api.kanye.rest")
.then((response: Superagent.Response) => {
this.quoteSlot.value = response.body.quote || DEFAULT_QUOTE;
});
}
render(h: IBlockHelper): React.ReactNode {
return (
<div tabIndex={h.tabIndex} className="kanye">
<blockquote>
<p className="quotation">
{this.quoteSlot.value || "..."}</p>
<footer>
— Kanye West
</footer>
</blockquote>
<div className={h.isActive ? "active" : ""}>
<button className="btn btn-lg btn-primary" onClick={() => h.next()}>
Continue
<i className="fas fa-level-down-alt fa-fw fa-rotate-90 ml-2" />
</button>
</div>
</div>
);
}
}
I’ve used Superagent to retrieve the quote from the API. React is used for updating the quote inside the paragraph tag. You can see that the slot is updated with the quote as soon it is retrieved. This way the quote is added to the response data and therefore stored when the respondent completes the survey. Add some Bootstrap styling and this results in a beautiful quote from Kanye West inside your survey 🕶️.
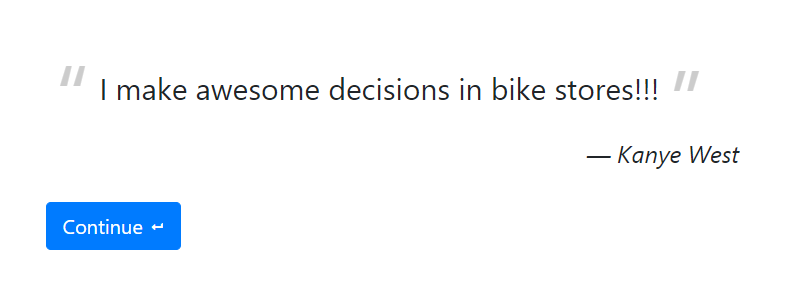
Try it yourself
You can experience the Kanye West block on GitLab Pages.
You can run this example on your local machine by downloading the source code from https://gitlab.com/tripetto/lab/kanye-west. Run npm install
and npm test
from your command prompt and you’re up and running on http://localhost:9000
.
Final thoughts
The Kanye West quote block is obviously meant for demo purposes (unless you are a real Kanye West fan and you want to share his wisdom with your target audience 😎). Imagine what you can do with custom blocks in your survey or even in your application. Tripetto can be fully integrated in your own software and its blocks are fully extendable as seen in this example.
Let me know what custom blocks you expect to create for your surveys.
Enjoy Tripetto!
(And very special thanks to @andrewjazbec for his Kanye Rest API!)